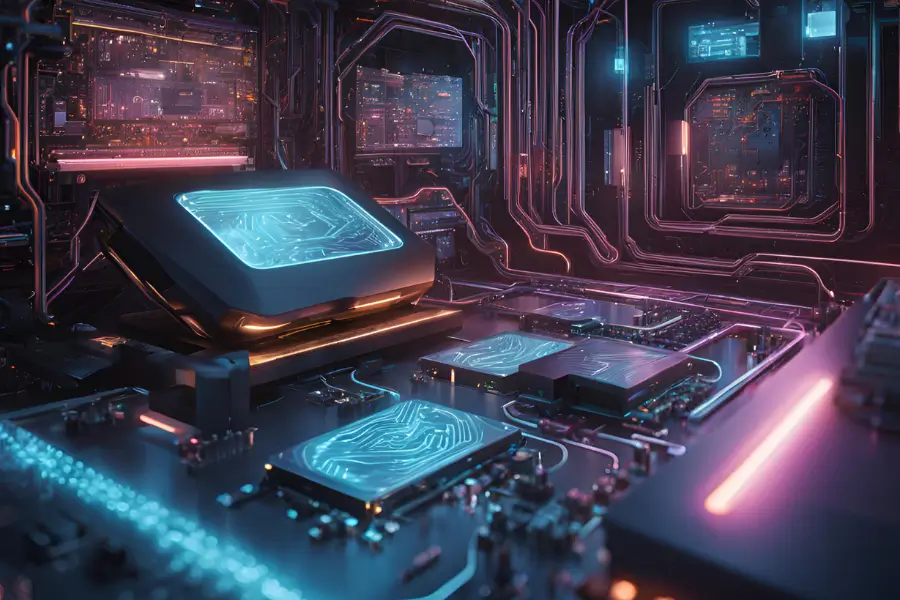
The world of artificial intelligence has been revolutionized by Convolutional Neural Networks (CNNs), a powerful deep learning technique that’s transforming how we approach image recognition and computer vision tasks. For beginners eager to dive into this cutting-edge field, implementing CNNs with TensorFlow is an essential first step. In this comprehensive guide, we’ll walk you through the process of building, training, and optimizing your very own CNN model using TensorFlow, Google’s open-source machine learning framework.
Whether you’re a curious student, an IT professional looking to expand your skillset, or an AI enthusiast ready to explore the depths of deep learning, this tutorial will open the door to the fascinating realm of neural networks and image processing. So, let’s embark on this exciting journey into the heart of modern machine learning!
Introduction to CNNs and TensorFlow
Convolutional Neural Networks (CNNs) represent a breakthrough in the field of artificial intelligence, particularly in computer vision tasks. Unlike traditional neural networks, CNNs are designed to automatically and adaptively learn spatial hierarchies of features from input images. This makes them incredibly effective for tasks such as image classification, object detection, and facial recognition.
TensorFlow, developed by Google, has quickly become one of the most popular frameworks for implementing deep learning models, including CNNs. Its flexibility, extensive documentation, and strong community support make it an excellent choice for both beginners and experienced practitioners.
Real-world applications of CNNs are all around us:
- Facial recognition systems in smartphones and security cameras
- Autonomous vehicles detecting road signs and pedestrians
- Medical imaging for disease diagnosis
- Content filtering on social media platforms
TensorFlow’s advantages for CNN implementation include:
- Efficient computation on both CPU and GPU
- Robust ecosystem with pre-built models and tools
- Seamless deployment options across various platforms
Setting Up Your Environment
Before we dive into building our CNN, let’s set up our development environment:
Install TensorFlow:
pip install tensorflow
For GPU support (recommended for faster training):
pip install tensorflow-gpu
Create a virtual environment:
python -m venv cnn_tutorial
source cnn_tutorial/bin/activate # On Windows use `cnn_tutorial\Scripts\activate`
Install additional dependencies:
pip install numpy matplotlib jupyter
Launch Jupyter Notebook:
jupyter notebook
Load the MNIST dataset:
from tensorflow.keras.datasets import mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
The MNIST dataset, containing 70,000 grayscale images of handwritten digits, is perfect for our beginner CNN project.
Building Your First CNN Model
Now, let’s construct our CNN architecture:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
Flatten(),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
Let’s break down this architecture:
- Conv2D layers: These apply convolutional filters to the input, learning spatial hierarchies of features.
- MaxPooling2D layers: These downsample the feature maps, reducing computational load.
- Flatten layer: This converts the 2D feature maps to a 1D vector for the final classification layers.
- Dense layers: These perform the final classification based on the learned features.
We’re using ReLU (Rectified Linear Unit) as our activation function for hidden layers due to its effectiveness in mitigating the vanishing gradient problem and promoting sparse activations.
Training and Evaluating Your CNN
With our model architecture defined, let’s compile and train it:
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
history = model.fit(x_train, y_train, epochs=10,
validation_data=(x_test, y_test))
Key hyperparameters to consider:
- Learning rate: Controlled by the Adam optimizer in this case
- Batch size: Default is 32, but you can experiment with different values
- Epochs: We’re using 10 here, but this can be adjusted based on your dataset and computational resources
To visualize our training progress:
import matplotlib.pyplot as plt
plt.plot(history.history['accuracy'], label='accuracy')
plt.plot(history.history['val_accuracy'], label='val_accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
This will give you a clear picture of how your model’s accuracy improves over time, and whether you’re overfitting or underfitting.
Tips for Improving Your CNN
To take your CNN to the next level, consider these advanced techniques:
Data Augmentation:
from tensorflow.keras.preprocessing.image import ImageDataGenerator
datagen = ImageDataGenerator(
rotation_range=10,
zoom_range=0.1,
width_shift_range=0.1,
height_shift_range=0.1
)
Dropout for regularization:
model.add(Dropout(0.5))
Transfer Learning:
base_model = tf.keras.applications.MobileNetV2(input_shape=(224, 224, 3),
include_top=False,
weights='imagenet')
base_model.trainable = False
Learning Rate Scheduling:
from tensorflow.keras.callbacks import LearningRateScheduler
def scheduler(epoch, lr):
if epoch < 10:
return lr
else:
return lr * tf.math.exp(-0.1)
callback = LearningRateScheduler(scheduler)
These techniques can significantly improve your model’s performance and generalization ability.
Conclusion and Next Steps
Congratulations! You’ve successfully built, trained, and optimized your first CNN using TensorFlow. This is just the beginning of your deep learning journey. Here are some next steps to consider:
- Experiment with different architectures and hyperparameters
- Try your model on more complex datasets like CIFAR-10 or ImageNet
- Explore advanced topics like object detection or semantic segmentation
- Dive into deployment strategies for your trained models
Remember, mastery in deep learning is an ongoing process. Keep experimenting, stay curious, and never stop learning. The field of AI is rapidly evolving, and your next project could be the one to push the boundaries of what’s possible with Convolutional Neural Networks!
By leveraging the power of TensorFlow and CNNs, you’re now equipped to tackle a wide range of computer vision challenges. So, fire up your GPU, start coding, and let’s see what amazing AI solutions you can create!